Making a game in 2 hours
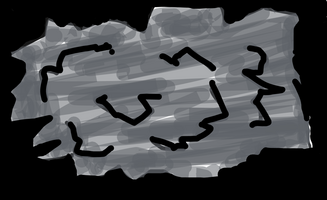
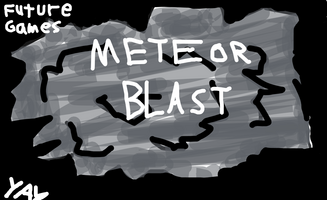
I made a game in 2 hours! A real, graphical game! AWESOME! ...How?
I worked on this game without a break for 2 hours. Here's how to try and fail at making a game.
Step 1: Idea
First find an idea for a simple game, or some inspiration. Yay, you're done with step 1.
Step 2: Design
Flesh up your idea. From "Click-blam Among Us task" to "Click to shoot at 3 randomly moving asteroids and get a high score in 30 seconds". Another short step.
Step 3: Graphics
Quickly make some simple graphics that work for your game. I made my graphics for this game in Chrome Canvas, but you can use anything. Tip--go 2D. This step may take a while.
Step 4: Sound effects
Don't prioritize this step. You can make sound effects and music in many programs. You can find plenty of free graphics on the internet, and if you pay, you can get better effects and even buy music for your game. I just Google "Free (blank) sound effects". Blank is the effect i need.
Step 5: Code
I spent 1.5 hours on code. My code is 4 kB. I know that sucks. But there were bugs, okay? And there will be bugs. This step is long, hard, and important. A game doesn't run without code. You may be using a game making tool like Scratch that has either block code or no code at all, but this tutorial is for HTML5 games. You will need to think "what do i need to do and how can i do it in a few minutes." Then get typing. Assuming you use HTML, your code may look like this:
<!DOCTYPE html>
<html>
<head>
<title>Meteor Blast</title>
<style>
body{
font-family: Courier New, monospace;
color: black;
background-color: #8B008B;
}
</style>
</head>
<body>
<canvas id="canvas" width="600" height="600" style="background-color:black"></canvas>
<script>
var can = document.getElementById("canvas");
var ctx = can.getContext('2d');
var score = 0;
var m1 = new Image();
var m2 = new Image();
var m3 = new Image();
m1.src = "resources/meteor.png";
m2.src = "resources/meteor.png";
m3.src = "resources/meteor.png";
var blam = new Audio();
blam.src = "resources/laser.mp3";
var time = 30;
var mX;
var mY;
var m1x;
var m1y;
var m2x;
var m2y;
var m3x;
var m3y;
var xs1;
var xs2;
var xs3;
var ys1;
var ys2;
var ys3;
window.addEventListener("mousemove", function(e){
mX = e.clientX;
mY = e.clientY;
});
function setup(){
m1x = 100;
m1y = 100;
m2x = 200;
m2y = 200;
m3x = 300;
m3y = 300;
xs1 = (Math.random() * 8) -4;
xs2 = (Math.random() * 8) -4;
xs3 = (Math.random() * 8) -4;
ys1 = (Math.random() * 8) -4;
ys2 = (Math.random() * 8) -4;
ys3 = (Math.random() * 8) -4;
}
function game(){
ctx.clearRect(0, 0, can.width, can.height);
ctx.drawImage(m1, m1x, m1y, 75, 75);
ctx.drawImage(m2, m2x, m2y, 75, 75);
ctx.drawImage(m3, m3x, m3y, 75, 75);
ctx.fillStyle = "white";
ctx.font = "22px monospace";
ctx.fillText(`Score: ${score}`, 10, 25);
ctx.fillText("Time: " + Math.ceil(time), 10, 50);
time -= 0.05;
m1x += xs1;
m1y += ys1;
m2x += xs2;
m2y += ys2;
m3x += xs3;
m3y += ys3;
if(m1x > 600)
m1x = 0;
if(m2x > 600)
m2x = 0;
if(m3x > 600)
m3x = 0;
if(m1x < 0)
m1x = 600;
if(m2x < 0)
m2x = 600;
if(m3x < 0)
m3x = 600;
if(m1y > 600)
m1y = 0;
if(m2y > 600)
m2y = 0;
if(m3y > 600)
m3y = 0;
if(m1y < 0)
m1y = 600;
if(m2y < 0)
m2y = 600;
if(m3y < 0)
m3y = 600;
window.addEventListener("click", function(){
blam.play();
if(mX > m1x && mX < m1x + 75 && mY > m1y && mY < m1y + 75){
score++;
m1x = Math.random() * 600;
xs1 = (Math.random() * 8) -4;
ys1 = (Math.random() * 8) -4;
}
if(mX > m2x && mX < m2x + 75 && mY > m2y && mY < m2y + 75){
score++;
m2x = Math.random() * 600;
xs2 = (Math.random() * 8) -4;
ys2 = (Math.random() * 8) -4;
}
if(mX > m3x && mX < m3x + 75 && mY > m3y && mY < m3y + 75){
score++;
m3x = Math.random() * 600;
xs3 = (Math.random() * 8) -4;
ys3 = (Math.random() * 8) -4;
}
});
if(time > 0){
window.setTimeout("game()", 50);
} else{
alert(`Game over! Final score: ${score}`);
window.location = "";
}
}
setup();
game();
</script>
</body>
</html>
That was basic. 150 lines. Yours may be better. The point is, you need to group everything that needs to happen, every mechanic, into functions. Then write each function, making them as independent from other functions as possible. Try to make short, efficient functions. And don't make too many.
Step 6: Bug fixing & playtesting
You're done! Playtest, bug fix, and your game is complete! Yay!
Files
Get Meteor Blast
Meteor Blast
Click goes blam
Status | Released |
Author | firet |
Genre | Shooter |
Tags | 2D, Arcade, bad, made-in-2-hours, Retro, Singleplayer |
Languages | English |
Accessibility | Color-blind friendly, One button, Textless |
More posts
- The game is released!Oct 13, 2020
Leave a comment
Log in with itch.io to leave a comment.